Data Structures & Algorithms Mastery
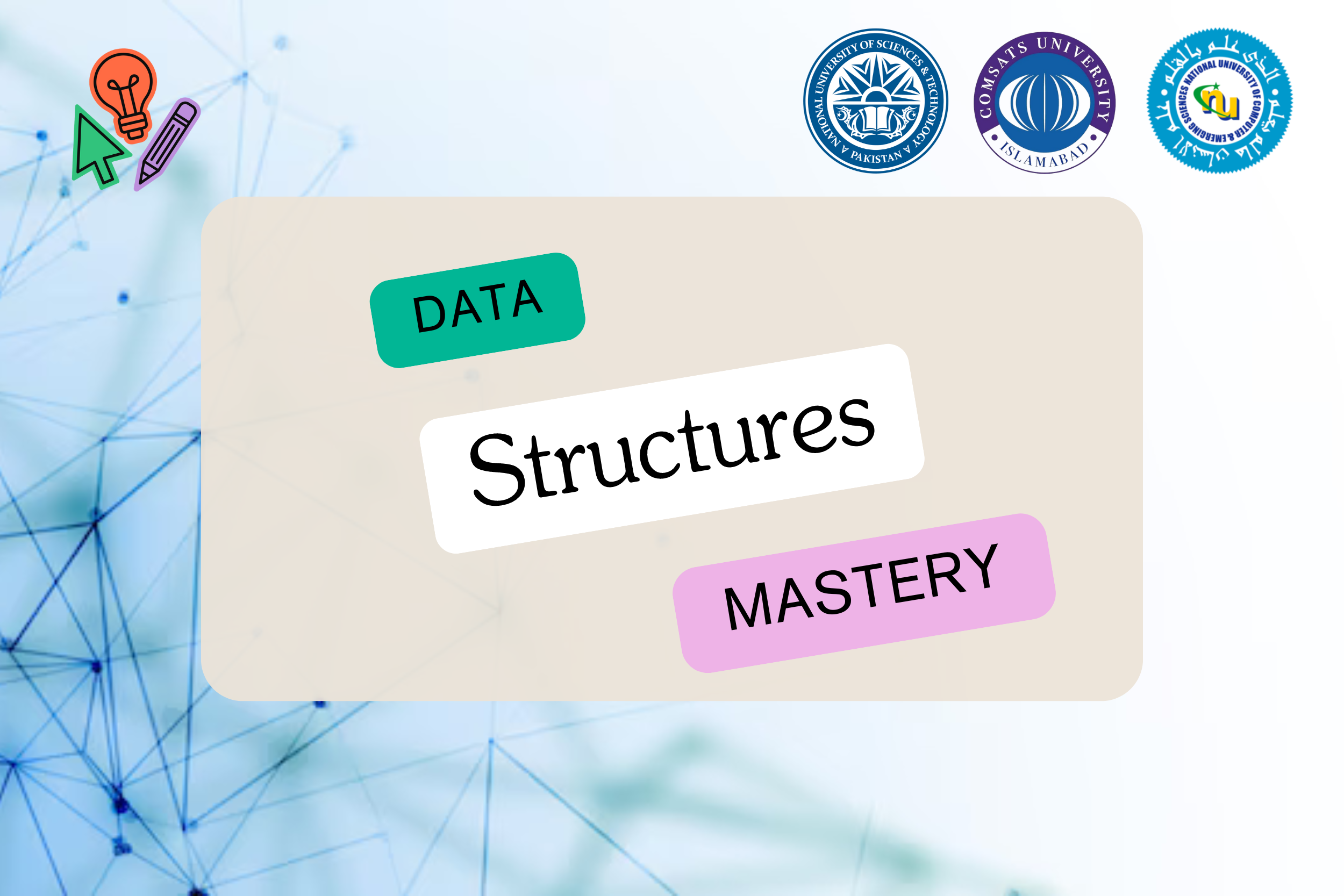
Course Outline: Data Structures and Algorithms
Course Description
The Data Structures and Algorithms course is designed for beginners to gain a solid understanding of fundamental data structures and algorithms, focusing on their implementation and application in programming. Over one month, with 12 lectures, students will explore various data structures, such as arrays, linked lists, stacks, queues, trees, and graphs. The course emphasizes algorithm design, complexity analysis, and practical problem-solving skills, using Java or C++. By the end of the course, students will be equipped to analyze and choose appropriate data structures and algorithms for different programming challenges.
What You’ll Learn From This Course
By the end of this course, students will:
- Understand the fundamental concepts of data structures and algorithms.
- Implement and utilize basic data structures such as arrays, linked lists, stacks, and queues.
- Analyze algorithm efficiency using Big O notation.
- Explore tree data structures and their applications.
- Understand graph theory, including graph representations and traversal algorithms.
- Apply sorting and searching algorithms in practical scenarios.
- Solve programming problems using appropriate data structures and algorithms.
Course Outline (Contents and Division)
Total Duration: 1 Month
Total Lectures: 12
Week 1 (Lectures 1-3)
- Lecture 1: Introduction to Data Structures
- Definition and importance of data structures.
- Overview of different types of data structures.
- Lecture 2: Arrays and Strings
- Introduction to arrays: single-dimensional and multi-dimensional.
- Basic operations: insertion, deletion, and traversal.
- Strings as character arrays and string manipulation.
- Lecture 3: Linked Lists
- Introduction to linked lists: singly and doubly linked lists.
- Basic operations: insertion, deletion, and traversal.
Week 2 (Lectures 4-6)
- Lecture 4: Stacks
- Introduction to stacks: LIFO concept.
- Operations: push, pop, peek, and implementation using arrays and linked lists.
- Lecture 5: Queues
- Introduction to queues: FIFO concept.
- Operations: enqueue, dequeue, and circular queues.
- Lecture 6: Recursion and Backtracking
- Understanding recursion: base case and recursive case.
- Problem-solving using recursion and backtracking techniques.
Week 3 (Lectures 7-9)
- Lecture 7: Trees
- Introduction to trees: binary trees and binary search trees (BST).
- Basic operations: insertion, deletion, and traversal (in-order, pre-order, post-order).
- Lecture 8: Heaps
- Introduction to heaps: min-heap and max-heap.
- Heap operations: insertion and deletion.
- Lecture 9: Hashing
- Introduction to hashing and hash tables.
- Collision resolution techniques: chaining and open addressing.
Week 4 (Lectures 10-12)
- Lecture 10: Introduction to Graphs
- Definition and representation of graphs: adjacency matrix and adjacency list.
- Types of graphs: directed, undirected, weighted, and unweighted.
- Lecture 11: Graph Traversal Algorithms
- Depth-First Search (DFS) and Breadth-First Search (BFS) algorithms.
- Applications of graph traversal.
- Lecture 12: Sorting and Searching Algorithms
- Introduction to sorting algorithms: bubble sort, selection sort, and insertion sort.
- Searching algorithms: linear search and binary search.
- Complexity analysis of sorting and searching algorithms.
Certification
Upon successful completion of the Data Structures and Algorithms course, students will receive a Certificate of Completion. This certificate signifies that the student has acquired essential knowledge and practical skills in data structures and algorithms, equipping them to tackle programming challenges effectively.